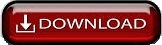

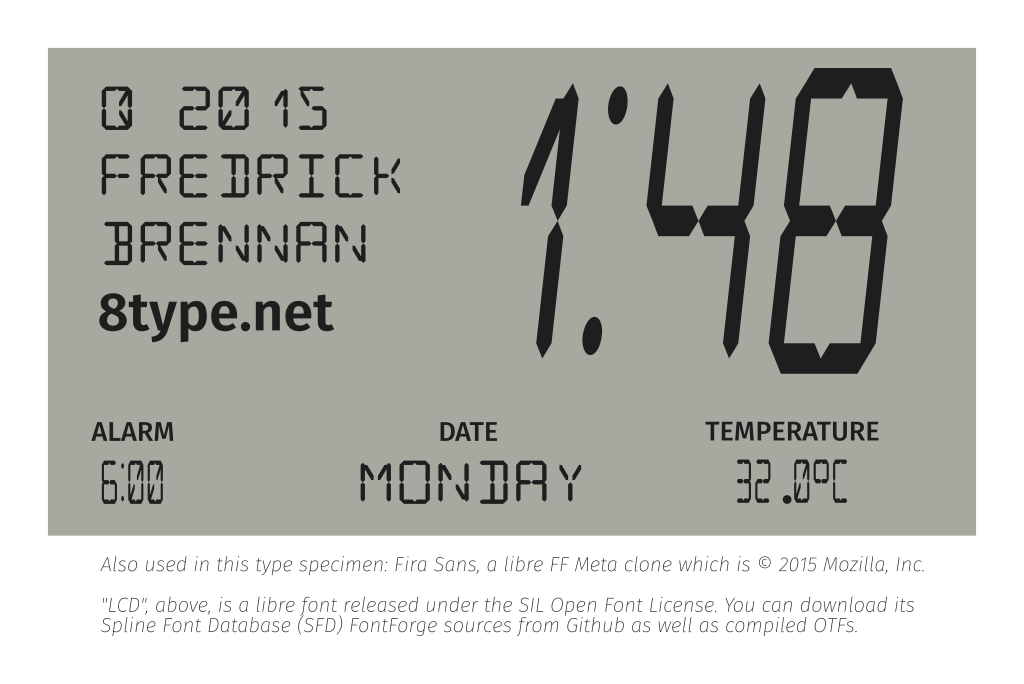
The for loop here is doing something really neat with only one line of code: Import registerClass import onionGpio import time # class to control a 7-segment display class SevenSegment: # class attribute # dictionary mapping each alphanumeric character to the bytestring that should be sent to the shift register digitMap = def _main_(): # if script was called with no arguments if len(sys.argv) = 1: print errorMsgs return -1 # if script was called with too many arguments elif len(sys.argv) > 2: print errorMsgs return -1 # read the hex string from the argument # extract the digits following the 0x # convert any uppercase letters to lowercase inputHexString = sys.argv inputHexString = inputHexString.split( "x") inputHexString = inputHexString.lower() inputLen = len(inputHexString) # validate the string's length if inputLen 4: print errorMsgs return -1 # check if valid hex digits (09, a-f) try: int(inputHexString, 16) except ValueError: print errorMsgs return -1 # attempt to display out the hex string while True: for i in range(inputLen): sevenDisplay.showDigit(i,inputHexString) # for running from the command line if _name_ = '_main_': _main_() This is usually how 7-segs are incorporated into a project in order to minimize the number of pins used on the controller.ħ-segment displays are sometimes called “7-segs” for short. This can really add up, so we can use a shift register to increase the number of output pins available to us. And in order to control multiple digits at once, we need one additional GPIO for each scan pin. To control the segments on a single-digit 7-seg display, you need at least seven GPIOs. This pin then controls whether the digit’s LEDs can be turned on or not. This isn’t really useful, so each digit has its own scan pin that groups segments for each digit together. For example, if you try to light up the segments will display a “1”, it will appear on all of the digits at the same time! See the diagram below for a typical labelling scheme:ħ-segment displays with more than one digit usually have each segment connected in parallel across all of the digits this is to save pins on the display module itself. Each segment is connected directly to an input pin and is controlled individually. If you take a look at a 7-segment display closely you’ll see that each digit is split into seven different segments, each lit up by an LED. If you’re wondering why it’s called a 7-segment display, wonder no more!
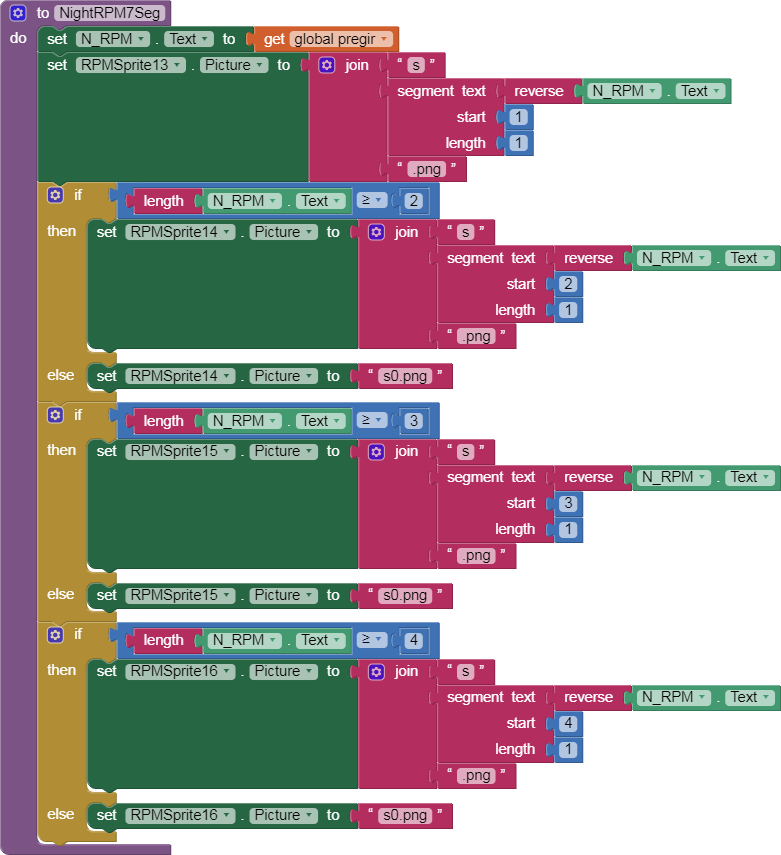
Using a Shift Register to Control Multiple LEDs.Unboxing and Getting the Hardware Ready.
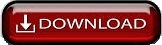